Introduction
This tutorial will walk you through creating a Python script to access Aligni’s GraphQL API and list all manufacturers that belong to your Aligni organization.
The script will be using GQL library for Python for easier access.
To make the script more interesting, you will modify it to also list all vendors for each manufacturer.
Prerequisites
To complete the tutorial you need:
- An Aligni account that can access an organization.
- A local Python development environment set up on your computer.
Process
Step 1 – Creating a New Python Project
Create a new directory by executing the following command in a terminal:
$ mkdir aligni_api_explorer
Enter the new directory:
$ cd aligni_api_explorer
Install GQL:
$ pip install gql
You should see that the new library was successfully installed:
Defaulting to user installation because normal site-packages is not writeable
Collecting gql
Downloading gql-3.5.0-py2.py3-none-any.whl (74 kB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 74.0/74.0 KB 1.1 MB/s eta 0:00:00
Collecting backoff<3.0,>=1.11.1
Downloading backoff-2.2.1-py3-none-any.whl (15 kB)
Collecting graphql-core<3.3,>=3.2
Downloading graphql_core-3.2.3-py3-none-any.whl (202 kB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 202.9/202.9 KB 4.5 MB/s eta 0:00:00
Collecting yarl<2.0,>=1.6
Downloading yarl-1.9.4-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (301 kB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 301.6/301.6 KB 10.9 MB/s eta 0:00:00
Collecting anyio<5,>=3.0
Downloading anyio-4.2.0-py3-none-any.whl (85 kB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 85.5/85.5 KB 17.3 MB/s eta 0:00:00
Collecting exceptiongroup>=1.0.2
Downloading exceptiongroup-1.2.0-py3-none-any.whl (16 kB)
Collecting sniffio>=1.1
Downloading sniffio-1.3.0-py3-none-any.whl (10 kB)
Collecting typing-extensions>=4.1
Downloading typing_extensions-4.9.0-py3-none-any.whl (32 kB)
Requirement already satisfied: idna>=2.8 in /usr/lib/python3/dist-packages (from anyio<5,>=3.0->gql) (3.3)
Collecting multidict>=4.0
Downloading multidict-6.0.5-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (124 kB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 124.3/124.3 KB 33.0 MB/s eta 0:00:00
Installing collected packages: typing-extensions, sniffio, multidict, graphql-core, exceptiongroup, backoff, yarl, anyio, gql
Successfully installed anyio-4.2.0 backoff-2.2.1 exceptiongroup-1.2.0 gql-3.5.0 graphql-core-3.2.3 multidict-6.0.5 sniffio-1.3.0 typing-extensions-4.9.0 yarl-1.9.4
Create a new file in the aligni_api_explorer directory called list_manufacturers.py in your editor of choice.
Add importing of required libraries to list_manufacturers.py:
import json
from gql import gql, Client
from gql.transport.requests import RequestsHTTPTransport
Step 2 – Obtain the Aligni API Token
To access the API, you need to have an API token. To get the token, log in to Aligni and visit Account Settings:
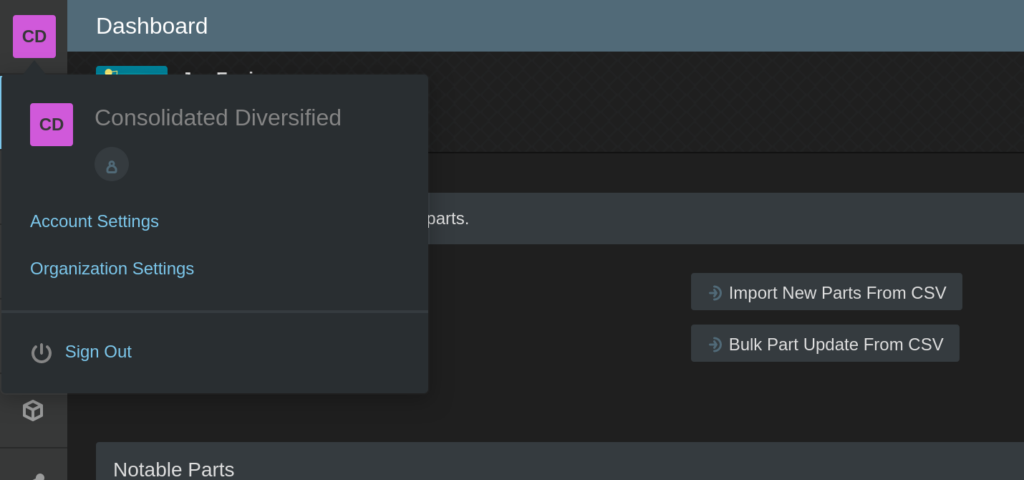
Then visit the API Tokens page:
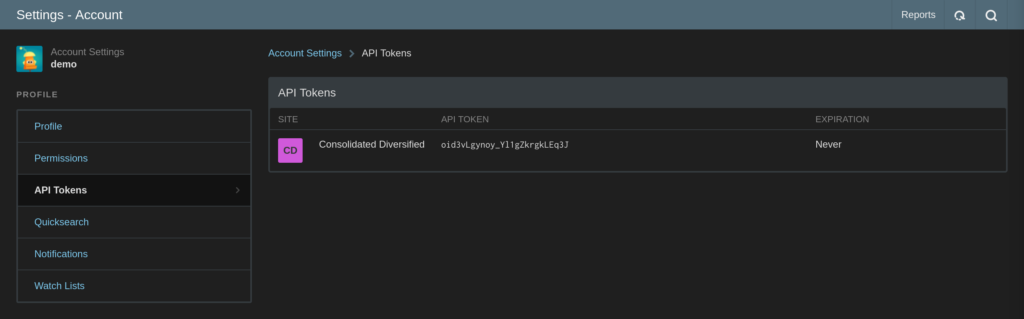
If this is the first time you’re using the API, you first need to generate a new token by clicking on the “Generate New Token” button.
Copy the new API token value and add it to your Python script:
auth_token = "oid3vLgynoy_Yl1gZkrgkLEq3J"
Step 3 – Prepare Creating a Request
Add the API endpoint URL to the script:
graphql_endpoint = "https://demo.aligni.org/api/v3/graphql"
Note: Replace the URL to the demo application (demo.aligni.org) with the URL to your Aligni organization (for example your-company.aligni.com).
Then, instantiate a client that you will use to communicate with the GraphQL API:
# Create a transport to the GraphQL endpoint with the "Authentication" header
transport = RequestsHTTPTransport(
url=graphql_endpoint,
use_json=True,
headers={"Authorization": f"Token {auth_token}"}
)
# Create a GraphQL client with the defined transport
client = Client(transport=transport, fetch_schema_from_transport=True)
Note that this code is using the URL that you previously defined and the API token value.
Step 4 – Add a Query For Listing Manufacturers
Next, add a GraphQL query for listing manufacturers that belong to your Aligni organization to the script:
# Define your GraphQL query
graphql_query = gql('''
query {
manufacturers {
nodes {
id
name
}
}
}
''')
This query is using the GraphQL query language to describe the data that you want to fetch.
Step 5 – Make the Request
The final step is to make the request. Add the code for making a request to the script:
# Make the GraphQL request using the client
response = client.execute(graphql_query)
# Print the response in a nice format
formatted_response = json.dumps(response, indent=2)
print(formatted_response)
This code makes the request and prints results in a human-readable format.
Step 6 – Run the Script
The whole script should look like this (with your API token value):
import json
from gql import gql, Client
from gql.transport.requests import RequestsHTTPTransport
auth_token = "oid3vLgynoy_Yl1gZkrgkLEq3J"
graphql_endpoint = "https://demo.aligni.org/api/v3/graphql"
# Create a transport to the GraphQL endpoint with the "Authentication" header
transport = RequestsHTTPTransport(
url=graphql_endpoint,
use_json=True,
headers={"Authorization": f"Token {auth_token}"}
)
# Create a GraphQL client with the defined transport
client = Client(transport=transport, fetch_schema_from_transport=True)
# Define your GraphQL query
graphql_query = gql('''
query {
manufacturers {
nodes {
id
name
}
}
}
''')
# Make the GraphQL request using the client
response = client.execute(graphql_query)
# Print the response in a nice format
formatted_response = json.dumps(response, indent=2)
print(formatted_response)
Now, open the terminal that you used to create the project directory and run the script:
$ python list_manufacturers.py
The script will list manufacturers that belong to your Aligni organization:
{
"manufacturers": {
"nodes": [
{
"id": "mfr_011XZV3AERGMRM87RHGQ48YSA2",
"name": "Alpha Wire Company"
},
{
"id": "mfr_011WVJ234GAV28NZVV743EKJNM",
"name": "AMP / Tyco"
},
{
"id": "mfr_011WVJ243RTTP8JSY87K9YADXS",
"name": "Amphenol"
},
{
"id": "mfr_011WVJ234G790WVBY0BY6DV1CR",
"name": "Analog Devices"
},
{
"id": "mfr_011WVJ243RQ8TXAJA3QRE1G8KZ",
"name": "API Delevan"
},
{
"id": "mfr_011XZT2JKG8XX5WAJ7WSEP02CD",
"name": "Atmel"
},
{
"id": "mfr_011WVJ234GAJPA9JNPYF04VPW6",
"name": "Bourns"
},
{
"id": "mfr_011WVJ243RP8WGN22ZD9715MJQ",
"name": "Consolidated Diversified"
},
{
"id": "mfr_011WVJ243RWN02QDGC3GAHB079",
"name": "CUI Inc."
},
{
"id": "mfr_011WVJ243R6VJPFADK3DH80WHQ",
"name": "Cypress Semiconductor"
}
]
}
}
Step 7 – Update the Query to List Vendors
Now, update the GraphQL query to list vendors for each manufacturer:
graphql_query = gql('''
query {
manufacturers {
nodes {
id
name
vendors {
nodes {
id
name
}
}
}
}
}
''')
Run the script again:
$ python list_manufacturers.py
And you will see the list of all manufacturers with the list of all vendors for each manufacturer:
{
"manufacturers": {
"nodes": [
{
"id": "mfr_011XZV3AERGMRM87RHGQ48YSA2",
"name": "Alpha Wire Company",
"vendors": {
"nodes": [
{
"id": "ven_011WVJ243R94FGAGYRCFC5GXK1",
"name": "Digi-Key"
}
]
}
},
{
"id": "mfr_011WVJ234GAV28NZVV743EKJNM",
"name": "AMP / Tyco",
"vendors": {
"nodes": [
{
"id": "ven_011WVJ243RD9SPKEZS736T9KER",
"name": "Arrow"
},
{
"id": "ven_011WVJ243RX2JHYBAZGBMYARM6",
"name": "Avnet"
},
{
"id": "ven_011WVJ243R94FGAGYRCFC5GXK1",
"name": "Digi-Key"
},
{
"id": "ven_011WVJ243R8B0VJV2AD8SFRHWV",
"name": "Initech Distributing"
},
{
"id": "ven_011WVJ243RH7J4V40RQST6A6K3",
"name": "Intertrode Global"
},
{
"id": "ven_011WVJ243RKZJFFTZB35S62KPP",
"name": "Mouser"
}
]
}
},
...
]
}
}